Blog
CLI and TUI programs
I wrote a document about my most loved and used CLI and TUI programs some time ago.
If you love to work in terminal emulators the document may be of interest to you... ;)
I love this UNIX world so much... ❤️
This is how my terminal workspace actually looks like:
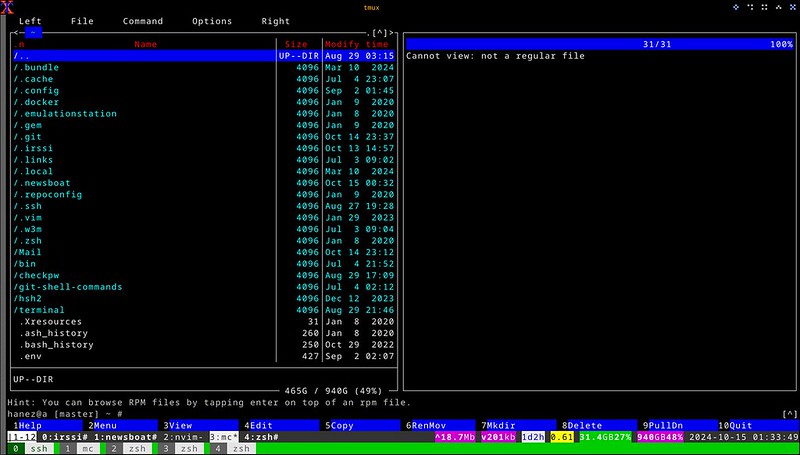
Permalink: https://hanez.org/2024/10/15/cli-and-tui-programs/
2023
Permalink: https://hanez.org/2023/12/16/2023/
Passkey... now with touch button!
My Passkey project got an update. I am using a touch based button now. The code is all the same as before but Passkey is more comfortable to use now. Take a look at my first first post about Passkey for more information.
More information will follow ASAP. I want to build a final device from these parts now... Maybe with a 3D printed enclosure to make it more professional... ;)
[UPDATE]: Renamed the project repository from "passkey" to "Passkey".
Permalink: https://hanez.org/2023/07/23/passkey-now-with-touch-button/
The Embedded Winter Challenge
I got some embedded devices in the past weeks and are starting playing around with hardware since many years again. I do this just for fun, but I really build some useful devices sometimes. Take a look at my blog post about Passkey to see what I actually do.
Devices from the top left to the bottom right:
- RFID-RC522 SPI based RFID Reader
- MicroSD SPI based Card Reader
- MCP2515 SPI based CAN Bus Shield
- Raspberry Pi Pico RP2040 microcontroller device running MicroPython
- Arduino Nano ATMega328 based device
- OLED I²C based Display with SSD1306 Chip 128 x 64 Pixel
- OLED I²C based Display Module 128 x 32 Pixel
- Arduino Leonardo Pro Micro ATmega32U4 based device
- Digispark ATTiny85 based device with micro USB port using the micronucleus bootloader
- Digispark ATTiny85 based device with USB-A connector using micronucleus
- RP2040-Zero Pico-Like MCU Board based on Raspberry Pi RP2040 microcontroller
- CP2102 micro USB to UART TTL Module
- AT24C256 I²C 256KB EEPROM memory
I own all of these devices more then once so the devices shown above do not all have pin headers soldered on them. It was looking nicer on the photo without headers... ;)
I am getting some more I²C devices in the next days! I am focussing on I²C devices because the I²C protocol is so easy to use, but SPI is interesting too.
For simple stuff I am using Arduino based devices, but I really like RP2040 based devices because I am very familiar with Python and running MicroPython makes so much sense to me. Also I like the possibility to run a REPL to interact with hardware interactively like a command shell. This is a lot of fun and debugging is a lot more easy too.
Permalink: https://hanez.org/2022/12/23/the-embedded-winter-challenge/
The UNIX System
I got this historical book "The UNIX System" by Stephen R. Bourne from 1983 today! I am impressed. It looks like it is new and it is nearly as old as I am... I always wanted to know more about the good old UNIX days so here I have a book which I believe will be very interesting to read.